Gottox/socket.io-java-client · GitHub
Socket.IO-Client for Java
socket.io-java-client is an easy to use implementation of socket.io for Java.
It uses Weberknecht as transport backend, but it's easy to write your own transport. See description below. An XHR-Transport is included, too. But it's not functional in its current state.
The API is inspired by java-socket.io.client.
Features:
- transparent reconnecting - The API cares about re-establishing the connection to the server when the transport is interrupted.
- easy to use API - implement an interface, instantiate a class - you're done.
- output buffer - send data while the transport is still connecting. No problem, socket.io-java-client handles that.
- meaningful exceptions - If something goes wrong, SocketIO tries to throw meaningful exceptions with hints for fixing.
Status: Connecting with Websocket is production ready. XHR is in beta.
How to use
Using socket.io-java-client is quite simple. But lets see:
Checkout and compile the project:
git clone git://github.com/Gottox/socket.io-java-client.git
cd socket.io-java-client
ant jar
mv jar/socketio.jar /path/to/your/libs/project
If you're using ant, change your build.xml to include socketio.jar. If you're eclipse, add the jar to your project buildpath.
Afterwards, you'll be able to use this library:
SocketIO socket = new SocketIO("http://127.0.0.1:3001/");
socket.connect(new IOCallback() {
@Override
public void onMessage(JSONObject json, IOAcknowledge ack) {
try {
System.out.println("Server said:" + json.toString(2));
} catch (JSONException e) {
e.printStackTrace();
}
}
@Override
public void onMessage(String data, IOAcknowledge ack) {
System.out.println("Server said: " + data);
}
@Override
public void onError(SocketIOException socketIOException) {
System.out.println("an Error occured");
socketIOException.printStackTrace();
}
@Override
public void onDisconnect() {
System.out.println("Connection terminated.");
}
@Override
public void onConnect() {
System.out.println("Connection established");
}
@Override
public void on(String event, IOAcknowledge ack, Object... args) {
System.out.println("Server triggered event '" + event + "'");
}
});
// This line is cached until the connection is establisched.
socket.send("Hello Server!");
For further informations, read the Javadoc.
Checkout
-
with git
git clone git://github.com/Gottox/socket.io-java-client.git
-
with mercurial
hg clone https://bitbucket.org/Gottox/socket.io-java-client
Both repositories are synchronized and up to date.
Building
to build a jar-file:
cd $PATH_TO_SOCKETIO_JAVA ant jar ls jar/socketio.jar
You'll find the socket.io-jar in jar/socketio.jar
Bugs
Please report any bugs feature requests to the Github issue tracker
Frameworks
This Library was designed with portability in mind.
- Android is fully supported.
- JRE is fully supported.
- GWT does not work at the moment, but a port would be possible.
- Java ME does not work at the moment, but a port would be possible.
- ... is there anything else out there?
Testing
There comes a JUnit test suite with socket.io-java-client. Currently it's tested with Eclipse.
You need node installed in PATH.
- open the project with eclipse
- open tests/io.socket/AllTests.java
- run it as JUnit4 test.
netty-socketio使用namespace - 烟火_ - 博客园
一、简介
netty-socketio中的namespace可以用于区别在相同连接地址下的不同用户,当两个不同的用户打开同一个页面的时候,可以使用namespace用来标记不同用户。例如我们可以在用户中心页面动态的获取用户的消息数目。这里就可以使用到namespace。因为每个用户的id都是不一样的,我们可以使用id来标识每个用户的namespace。
二、示例
服务器端代码:
package com.test.socket; import com.corundumstudio.socketio.Configuration; import com.corundumstudio.socketio.SocketIONamespace; import com.corundumstudio.socketio.SocketIOServer; public class SocketServer2 { public static void main(String[] args) throws InterruptedException { Configuration config = new Configuration(); config.setHostname("localhost"); config.setPort(9092); final SocketIOServer server = new SocketIOServer(config); server.start(); String uid = "1111"; String namespace = String.format("/%s_%s", "msg", uid);//构建命名空间 SocketIONamespace chat1namespace = server.addNamespace(namespace); //设置命名空间 for (int i = 0; i < 50; i++) { Thread.sleep(2000); chat1namespace.getBroadcastOperations().sendEvent("message", 1); //每次发送数字一 } Thread.sleep(Integer.MAX_VALUE); server.stop(); } }
客户端message.html代码:
1 <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> 2 <html> 3 <head> 4 <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> 5 <title>Insert title here</title> 6 <script src="./jquery-1.9.1.js" type="text/javascript"></script> 7 <script type="text/javascript" src="./socket.io/socket.io.js"></script> 8 9 <style> 10 body { 11 padding:20px; 12 } 13 #console { 14 overflow: auto; 15 } 16 .username-msg {color:orange;} 17 .connect-msg {color:green;} 18 .disconnect-msg {color:red;} 19 .send-msg {color:#888} 20 </style> 21 22 </head> 23 24 <body> 25 26 <h1>Netty-socketio Demo Chat</h1> 27 28 <br/> 29 30 <div id="console" class="well"> 31 </div> 32 消息总数:<div id="msgnum">0</di> 33 </body> 34 35 36 37 <script type="text/javascript"> 38 var socket = io.connect('http://localhost:9092/msg_1111'); 39 40 socket.on('connect', function() { 41 output('<span class="connect-msg">Client has connected to the server!</span>'); 42 }); 43 44 socket.on('message', function(data) {//收到消息后,将消息总数加一 45 var num = $("#msgnum").html(); 46 num = parseInt(num) + data; 47 $("#msgnum").html(num); 48 }); 49 50 socket.on('disconnect', function() { 51 output('<span class="disconnect-msg">The client has disconnected!</span>'); 52 }); 53 function sendDisconnect() { 54 socket.disconnect(); 55 } 56 57 function output(message) { 58 var currentTime = "<span class='time'>" + new Date() + "</span>"; 59 var element = $("<div>" + currentTime + " " + message + "</div>"); 60 $('#console').prepend(element); 61 } 62 63 </script> 64 </html>
启动服务器,访问该网页,消息总数会每次加1。
#数据技术选型#即席查询Shib+Presto,集群任务调度HUE+Oozie - 旁观者 - 博客园
使用者是产品/运营/销售运营的数据分析师;要求数据分析师掌握查询SQL查询脚本编写技巧,掌握不同业务的数据存储在不同的数据集市里;不管他们的计算任务是提交给 数据库 还是 Hadoop,计算时间都可能会很长,不可能在线等待;所以,使用者提交了一个计算任务(PIG/SQL/Hive SQL),控制台告知任务已排队,给出大致的计算时间等友情提示, 这些作业的权重较低,使用者和管理员可以查看排队中的计算任务,包括已执行任务的执行时间、运行时长和运行结果;当计算任务有结果后,控制台界面有通知提示,或者发邮件提示,使用者可以在线查看和下载数据。
Presto 简化的架构如下图1所示,客户端将 SQL 查询发送到 Presto 的协调器。协调器会进行语法检查、分析和规划查询计划。调度器将执行的管道组合在一起,将任务分配给那些离数据最近的节点,然后监控执行过程。客户端从输出段中将数据取出,这些数据是从更底层的处理段中依次取出的。
Presto 的运行模型与 Hive 有着本质的区别。Hive 将查询翻译成多阶段的 Map-Reduce 任务,一个接着一个地运行。 每一个任务从磁盘上读取输入数据并且将中间结果输出到磁盘上。然而 Presto 引擎没有使用 Map-Reduce。它使用了一个定制的查询执行引擎和响应操作符来支持SQL的语法。除了改进的调度算法之外,所有的数据处理都是在内存中进行的。不同的处理端通过网络组成处理的流水线。这样会避免不必要的磁盘读写和额外的延迟。这种流水线式的执行模型会在同一时间运行多个数据处理段,一旦数据可用的时候就会将数据从一个处理段传入到下一个处理段。
这样的方式会大大的减少各种查询的端到端响应时间。
同时,Presto 设计了一个简单的数据存储抽象层,来满足在不同数据存储系统之上都可以使用 SQL 进行查询。存储连接器目前支持除 Hive/HDFS 外,还支持 HBase、Scribe 和定制开发的系统。
图1. Presto架构
- Oozie允许失败的工作流从任意点重新运行,这对于处理工作流中由于前一个耗时活动而出现瞬态错误的情况非常有用。
- 工作流执行过程可视化。
- 工作流的每一步的日志、错误信息都可以点击查看,并实时滚动,便于排查问题。
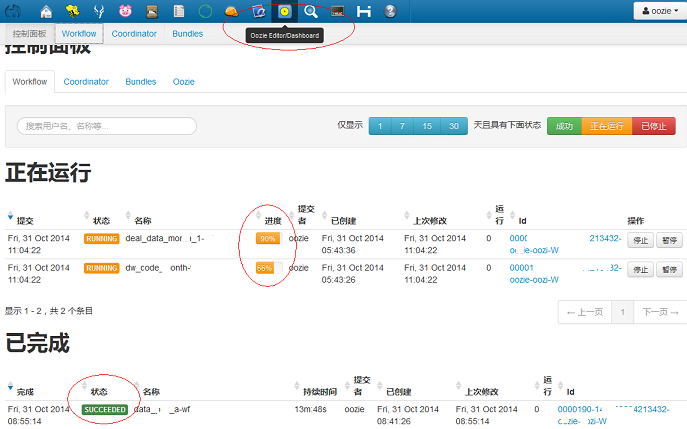
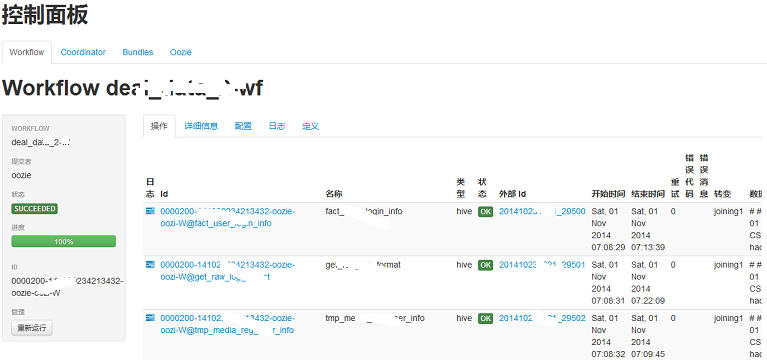
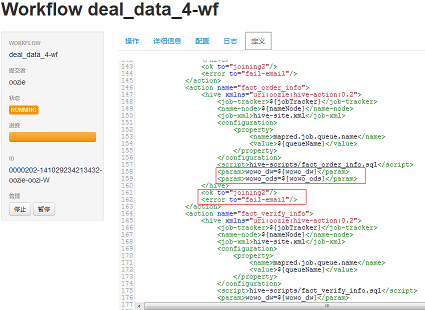